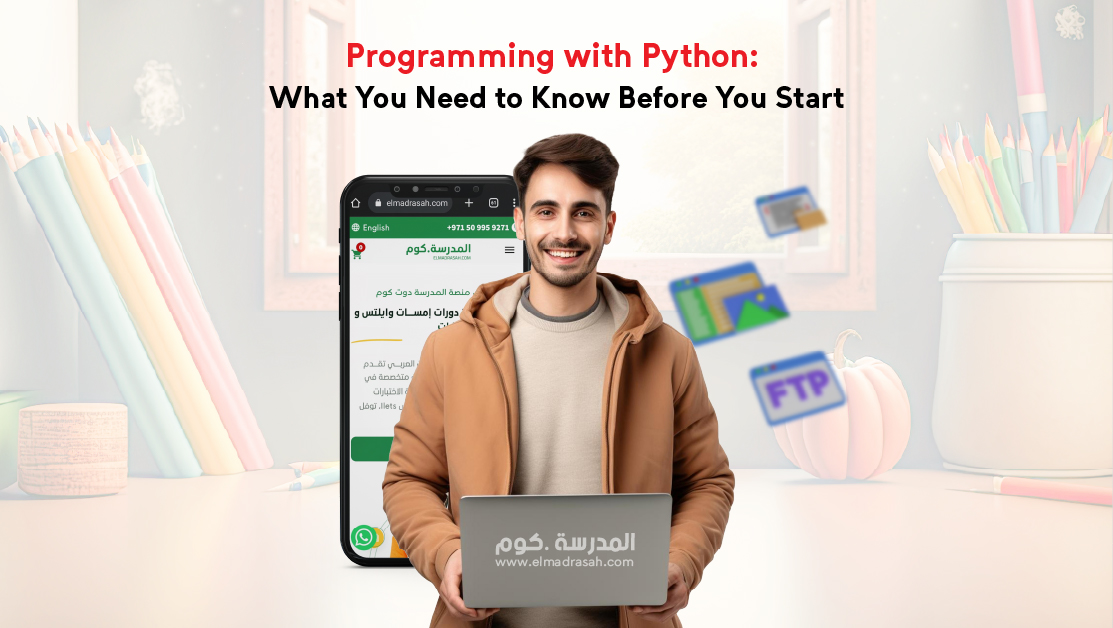
Python programming language is renowned for its ease of learning and versatile usage in a wide range of applications, making it one of the most popular and widely used languages in the programming world. If you’re considering starting to learn Python, it’s important to familiarize yourself with the fundamental concepts and important aspects you need to know before embarking on your journey into the programming world with this language. In this article, we’ll explore everything you need to know before starting programming with Python, including its advantages, uses, useful resources for learning, and more. If you’re looking to build a strong foundation in Programming with Python, then this article will be the perfect starting point for you.
Introduction to Python Programming
Python is one of the popular programming languages widely used across various fields from software development to data science and artificial intelligence. Python was developed in the late 1980s by Guido van Rossum and is considered one of the easiest and most understandable languages with powerful capabilities.
History and Evolution of Python
Python emerged from a simple and clear philosophy, aiming to create a programming language that is easy to use and readable, resembling the English language in expression. The first version of Python (version 0.9.0) was released in 1991, and since then, Python has experienced continuous development and tremendous growth.
Advantages of Using Python
Python boasts several advantages that have made it one of the most popular and widely used languages across many fields, including:
- Ease of use: Python is easy to learn and use, suitable for both beginners and professionals alike.
- Versatility: Python can be used in developing a wide variety of applications, including web development, desktop applications, data analysis, artificial intelligence applications, and more.
- Active community: Python has a large and active community of developers and users who provide support, assistance, and facilitate learning and development.
- Scalability: Python can easily scale and evolve to meet the needs of growing projects.
- Rich libraries: Python comes with extensive and rich libraries that help accelerate the development process and provide ready-made solutions to many common problems.
Common Uses of Python
Python is used in a variety of applications and fields, including:
- Web development using the Django framework.
- Desktop application development using libraries such as PyQt or Tkinter.
- Scientific computing and mathematics using libraries like NumPy, SciPy, and Pandas.
- Development of artificial intelligence applications and machine learning using libraries such as TensorFlow or PyTorch.
Python is a powerful and versatile programming language used widely across many fields. If you’re considering learning a new programming language or starting a career in software development, Python might be an ideal choice for you, whether you’re a beginner or a seasoned programmer.
Python Applications in Various Fields
Python is one of the most popular and widely used programming languages in the world today. This is partly due to its ease of learning and use, as well as its power and flexibility in development. Python can be used in a wide range of fields, including web and software applications, data science, and artificial intelligence. In this article, we’ll explore some common applications of Python in various fields.
- Web Development: Python provides several popular frameworks for web development such as Django and Flask. These frameworks are known for their ease of use and scalability, making Python a popular choice among developers for building websites and web applications.
- Data Analysis and Science: With powerful libraries like NumPy, Pandas, and Matplotlib, scientists and analysts use Python for organizing and analyzing data, creating visualizations, and applying machine learning and artificial intelligence techniques to data.
- Game Development: Python can be used for developing video games, whether independent or large-scale. With libraries like Pygame, developers can quickly and efficiently create amazing games using Python.
- Mobile Application Development: With frameworks like Kivy, Python can be used to develop mobile applications across multiple platforms such as iOS and Android. This allows developers to build a single application that runs on multiple operating systems.
- Engineering and Design: Engineers and designers use Python for creating models, simulations, and data analysis in fields such as mechanical engineering, architectural design, and 3D graphics.
- Robotics and Automation: Using frameworks like Robot Framework and Raspberry Pi, Python can be used for developing robotics and automation applications. Python is used for controlling robots, building remote sensing and control systems, and many other applications in the field of robotics and automation.
These are just a few examples showcasing the diversity of Python usage in various fields. With its ease of learning and use, Python provides exciting opportunities for developers, engineers, and researchers to create innovative applications in different domains.
Environments and Tools Used in Python Development
Python is one of the most widely used programming languages in the world, offering many environments and tools for application development. In this article, we’ll explore some popular development environments and useful tools used by developers in Python application development.
Integrated Development Environments (IDEs):
- PyCharm: PyCharm is one of the most popular integrated development environments for Python development. PyCharm provides powerful features such as code completion, automatic error correction, code analysis, and effective project organization.
- Visual Studio Code: Visual Studio Code (VSCode) is a powerful and lightweight development environment that fully supports Python. VSCode offers advanced editing features and a wide range of extensions for customizing the environment to meet developers’ needs.
- Jupyter Notebook: Jupyter Notebook is used for interactive development, experimentation, and code documentation. It is particularly useful in data science and machine learning, allowing users to add explanatory texts and graphics to the code.
Text Editors:
- Sublime Text: Sublime Text provides a simple and lightweight user interface, known for its speed and customization capabilities. It is a good option for text editing and programming with Python.
- Atom: Atom offers powerful editing features and a wide range of plugins for customizing the environment to meet developers’ needs. Atom can be easily used for developing Python applications.
Environment Management Tools:
- Anaconda: Anaconda is used for managing virtual environments and installing libraries and tools related to data science and software development. Anaconda provides an easy-to-use interface for managing projects using Python.
- Pipenv: Pipenv is used to create and manage Python environments and install libraries in an organized manner. Pipenv combines package and environment management features into one tool.
Testing and Debugging Tools:
- Pytest: Pytest is a powerful and easy-to-use testing framework for testing Python applications. Pytest provides a clear testing interface and advanced features for controlling testing operations.
- pdb: pdb is a built-in debugging tool in Python used for running and debugging scripts interactively. pdb provides features for tracking execution and identifying problems in the code.
These are just some of the environments and tools used by developers in Python application development. The tools used depend on the developer’s needs and preferences, and each developer should choose the tool that best meets their project requirements. By using the right tools, developers can accelerate the development process and improve the efficiency of code written in the Python language.
Basic Concepts in Python: Variables, Strings, and Lists
Python is a powerful and easy-to-learn programming language used in a wide range of applications, including web applications, data science, and artificial intelligence. In this article, we’ll explore some basic concepts in Python, namely variables, strings, and lists.
Variables in Python:
Variables in Python are symbols used to store values in memory. Variables can contain different types of data such as numbers, strings, lists, dates, and more. Variables in Python are created by writing the variable name followed by the assignment operator “=” and then the value. For example:
x = 5 # a variable containing the number 5
name = “John” # a variable containing the string “John”
Strings in Python:
Strings in Python are sequences of characters enclosed in quotation marks (“) or (‘). Strings can be used to represent fixed texts such as messages, names, or any other text. Various operations can be performed on strings such as concatenation, splitting, and extraction. For example:
message = “Hello, world!” # a string containing the greeting “Hello, world!”
Lists in Python:
Lists in Python are ordered collections of elements that can be accessed, modified, and removed. Lists are defined by placing the elements within square brackets [] and separating them with commas. Lists can contain elements of different data types such as numbers, strings, and even other lists. For example:
numbers = [1, 2, 3, 4, 5] # a list of numbers
fruits = [“apple”, “banana”, “orange”] # a list of fruits
mixed_list = [1, “apple”, True, [2, 3, 4]] # a list containing different data types
Variables, strings, and lists play a crucial role in building Python programs. They can be used for storing data, analyzing data, organizing data, and many other functions. These basic concepts are the starting point for understanding the language and getting started with programming various applications using Python.
Organizational Structure in Python: Conditions and Loops
In software development, understanding the organizational structure in a programming language is crucial for writing programs effectively and efficiently. In Python, the basic organizational structures consist of conditions and loops, which allow for organizing the flow of program execution. Let’s take a deep dive into each of them.
Conditions in Python:
Conditions in Python allow the program to make specific decisions based on certain values. Conditions typically consist of comparison expressions that check whether certain expressions are true or not. In Python, keywords like `if`, `else`, and `elif` are used to specify conditions. For example:
“`python
x = 5
if x > 0:
print(“Positive”)
elif x == 0:
print(“Zero”)
else:
print(“Negative”)
“`
In this example, the value of the variable `x` is checked, and the result is printed according to the appropriate condition.
Loops in Python:
Loops in Python allow the program to execute a certain block of code repeatedly, facilitating dealing with repetitions and iterations in the program. Loops come in several forms in Python, including the `for` loop and the `while` loop. For example:
“`python
fruits = [“apple”, “banana”, “cherry”]
for fruit in fruits:
print(fruit)
“`
This example uses a `for` loop to iterate over the list of fruits and print each item in the list.
Common Usage Between Conditions and Loops:
Conditions and loops in Python are commonly used to organize program execution. Conditions can be used inside loops to make dynamic decisions based on the data being used. For example:
“`python
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number % 2 == 0:
print(f”{number} is even”)
else:
print(f”{number} is odd”)
“`
In this example, a `for` loop is used to iterate over the list of numbers, and the condition inside the loop is used to check whether the numbers are even or odd.
Conditions and loops are essential parts of the organisational structure in Python, allowing for organizing the program execution flow logically and systematically. By understanding how to use conditions and loops correctly, programmers can write powerful and efficient Python programs that effectively meet their needs.
Strategies for Effective Learning of the Python Language
Python is one of the famous and widely used programming languages in various fields such as web development, artificial intelligence, data science, and more. Since Python is considered an easy-to-learn and effective programming language, effective learning strategies play a crucial role in acquiring the necessary skills in this language. Here are some strategies and Tips for Learning Python Language that can help you learn Python better:
- Setting Goals: Before starting to learn Python, you should set goals that you want to achieve. Do you want to develop web applications? Or do you want to work in the field of data science? By setting goals, you can direct your efforts and focus on the concepts and skills you need.
- Starting with the Right Resources: There are many resources available for learning Python, such as books, online courses, and popular websites like Codecademy. Choose resources that suit your learning style and cover the topics you are interested in.
- Practical Application: Practical application is one of the most important strategies for effectively learning Python. Solve coding challenges, write small programs, and work on practical projects that help you apply the concepts you have learned in real-world contexts.
- Engaging in the Community: Join online programming communities such as Stack Overflow, Reddit, and Python forums. You can ask questions, share knowledge, and communicate with experienced programmers to get help and advice.
- Maintaining Consistency: Keep practicing consistency in learning Python and developing your skills. Allocate daily or weekly time for learning and application, and continue to develop your projects and solve coding problems.
- Creating Personal Projects: Create personal projects that allow you to apply what you have learned from Python in a practical context. Develop simple applications, tools, or even small games, which will help you improve your skills and increase your confidence in programming.
- Keeping Up with Developments: Python is a language that constantly evolves, so you should keep up with the latest developments and features in the language. Check technical blogs, official websites, and news sites to stay updated with everything new.
By using these strategies, you can enhance your learning experience in Python and make significant progress in acquiring the necessary skills for developing applications and programs based on this powerful and flexible programming language.
Future Directions for Continuing to Develop Python Skills
Python isn’t just a programming language; it’s a platform for creativity and innovation across various fields. If you’ve embarked on your journey to learn Python and develop your skills in this powerful language, future directions will help you continue your journey effectively and successfully.
Engage with the Programming Community:
Join various programming communities online and specialized forums dedicated to Python. This gives you the opportunity to interact with other programmers, exchange experiences, ask questions to troubleshoot problems, and engage in technical discussions.
Continue Learning Resources:
Keep following available learning resources for Python, such as specialized programming books, online courses, and technical articles. You can also enroll in advanced courses covering advanced topics in Python.
Participate in Practical Projects:
Engage in practical projects that utilize Python in real-world scenarios. This helps you apply the concepts you’ve learned in practical contexts and allows you to build a portfolio that reflects your programming skills and capabilities using Python.
Stay Updated with Technological Developments:
As Python is a dynamic and evolving programming language, it’s essential to stay updated with technological developments and new features added to the language. Check out technical blogs, tech news websites, and official Python sites to stay abreast of the latest advancements.
Continue Personal Development:
Continued development of Python skills shouldn’t just focus on technical skills but also on personal development. Improve communication, problem-solving, time management, and teamwork skills, which will help you succeed in the field of programming.
Search for Job Opportunities:
Look for job opportunities that require Python skills, whether they are permanent positions or freelance projects. Build a professional resume highlighting your Python skills and the projects you’ve worked on, and apply for jobs that align with your interests and skills.
Contribute to Open Source Projects:
Search for open-source projects that use Python and contribute to them. You can contribute by improving code, fixing bugs, adding new features, which will help you enhance your skills and learn best practices in programming.
By following these directions, you can continue to develop your Python skills and reach new levels of expertise and professionalism in this distinctive programming language.
The First Steps to Start Programming with Python
If you’re considering starting to learn programming and want to choose a language that’s easy to learn and powerful at the same time, Python might be the perfect choice for you. It’s important to follow certain steps to successfully begin your journey into the world of Programming with Python. In this article, we’ll take a look at the first steps you should take to start programming with Python.
- Install Python on Your Computer: The first step is to install Python on your computer. You can do this by visiting the official Python website online and downloading the latest available version for your operating system. After installing Python, you’ll be able to use it to run programs and write commands.
- Learn Programming Basics: Once you’ve installed Python, you should start learning the basics of programming. This includes understanding fundamental concepts like variables, functions, conditions, and loops. You can benefit from a wide range of online resources, such as tutorials, videos, and books, that explain these concepts in an easy-to-understand manner.
- Use an Integrated Development Environment (IDE): You can use an Integrated Development Environment (IDE) like PyCharm or Visual Studio Code to write and run Python programs more efficiently. These environments provide useful features such as smart editing, syntax highlighting, and automatic code formatting, making the programming process easier and smoother.
- Work on Small Projects: Once you’ve mastered the basics, you can start creating small projects using Python. These projects can be simple, such as a calculator program or an application that converts temperatures, and they help you apply the concepts you’ve learned in a practical context.
- Engage in the Programming Community: Don’t hesitate to engage in the programming community through online forums and social media groups. You can ask questions, share your projects, meet other developers, and benefit from their experiences and advice.
By following these basic steps, you can start your journey into the world of Programming with Python. Remember that persistence and perseverance are the keys to success, so enjoy learning and exploring Python’s limitless possibilities.
Learn Python Programming with elmadrasah.com Courses
In the modern era of technology, programming has become a fundamental skill for many jobs and fields, whether you’re a university student, a job seeker, or a professional in a specific field. Among the popular and preferred programming languages by programmers and developers worldwide, Python stands out for its simplicity and power.
Python: The Perfect Programming Language for Beginners
Python is known for its ease of learning and understanding, adopting an approach that focuses on clarity and simplicity in code writing. This means that individuals who are starting to learn programming for the first time may find Python to be the perfect choice for them. With the increasing demand for Python experts in the job market, mastering this language can be the key to unlocking many professional opportunities.
elmadrasah.com Courses: Your Key to Mastering Python
elmadrasah.com offers a diverse and comprehensive range of Python courses that cater to various skill levels and needs. Here are some reasons why elmadrasah.com Courses are the optimal choice for learning Python:
- Carefully Designed Courses: elmadrasah.com Courses are carefully designed to meet the needs of learners at all levels, from beginners to advanced, with the best private tutor for learning programming. Whether you want to understand the basics or advance in more complex concepts, there’s a suitable course for you.
- Comprehensive Educational Content: elmadrasah.com Courses include thorough and organised explanations of topics, providing practical examples and interactive exercises to ensure your full understanding of concepts and their practical application.
- Taught by Certified Professionals: In elmadrasah.com Courses, instructors provide high-quality education, with extensive experience in their fields and the necessary training to deliver educational materials effectively and engagingly.
- Flexible Schedule: elmadrasah.com Courses allow learners to access study materials anytime, anywhere, enabling you to deliver your lessons and pursue your learning flexibly and easily.
- Supportive Learning Community: elmadrasah.com’s learning community provides a supportive and motivating environment where you can share ideas and experiences with others, learning from their experiences and advice.
Prepare for the Future with Python and elmadrasah.com Courses
By using elmadrasah.com Courses, you can be prepared to face the challenges of the growing job market and achieve success in the field of Programming with Python. Start your educational journey today and join the learning community at elmadrasah.com to acquire the skills that will enable you to achieve your professional and personal goals.